mirror of
https://github.com/Vendicated/Vencord.git
synced 2024-09-19 22:20:34 +00:00
Compare commits
3 commits
6bc48ebe32
...
65aa55383d
Author | SHA1 | Date | |
---|---|---|---|
|
65aa55383d | ||
|
c7e5295da0 | ||
|
e4ec89e0ba |
5 changed files with 90 additions and 83 deletions
5
src/plugins/fullSearchContext/README.md
Normal file
5
src/plugins/fullSearchContext/README.md
Normal file
|
@ -0,0 +1,5 @@
|
|||
# FullSearchContext
|
||||
|
||||
Makes the message context menu in message search results have all options you'd expect.
|
||||
|
||||

|
82
src/plugins/fullSearchContext/index.tsx
Normal file
82
src/plugins/fullSearchContext/index.tsx
Normal file
|
@ -0,0 +1,82 @@
|
|||
/*
|
||||
* Vencord, a modification for Discord's desktop app
|
||||
* Copyright (c) 2023 Vendicated and contributors
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
import { migratePluginSettings } from "@api/Settings";
|
||||
import { Devs } from "@utils/constants";
|
||||
import definePlugin from "@utils/types";
|
||||
import { findByPropsLazy } from "@webpack";
|
||||
import { ChannelStore, ContextMenuApi, i18n, UserStore } from "@webpack/common";
|
||||
import { Message } from "discord-types/general";
|
||||
import type { MouseEvent } from "react";
|
||||
|
||||
const { useMessageMenu } = findByPropsLazy("useMessageMenu");
|
||||
|
||||
function MessageMenu({ message, channel, onHeightUpdate }) {
|
||||
const canReport = message.author &&
|
||||
!(message.author.id === UserStore.getCurrentUser().id || message.author.system);
|
||||
|
||||
return useMessageMenu({
|
||||
navId: "message-actions",
|
||||
ariaLabel: i18n.Messages.MESSAGE_UTILITIES_A11Y_LABEL,
|
||||
|
||||
message,
|
||||
channel,
|
||||
canReport,
|
||||
onHeightUpdate,
|
||||
onClose: () => ContextMenuApi.closeContextMenu(),
|
||||
|
||||
textSelection: "",
|
||||
favoriteableType: null,
|
||||
favoriteableId: null,
|
||||
favoriteableName: null,
|
||||
itemHref: void 0,
|
||||
itemSrc: void 0,
|
||||
itemSafeSrc: void 0,
|
||||
itemTextContent: void 0,
|
||||
});
|
||||
}
|
||||
|
||||
migratePluginSettings("FullSearchContext", "SearchReply");
|
||||
export default definePlugin({
|
||||
name: "FullSearchContext",
|
||||
description: "Makes the message context menu in message search results have all options you'd expect",
|
||||
authors: [Devs.Ven, Devs.Aria],
|
||||
|
||||
patches: [{
|
||||
find: "onClick:this.handleMessageClick,",
|
||||
replacement: {
|
||||
match: /this(?=\.handleContextMenu\(\i,\i\))/,
|
||||
replace: "$self"
|
||||
}
|
||||
}],
|
||||
|
||||
handleContextMenu(event: MouseEvent, message: Message) {
|
||||
const channel = ChannelStore.getChannel(message.channel_id);
|
||||
if (!channel) return;
|
||||
|
||||
event.stopPropagation();
|
||||
|
||||
ContextMenuApi.openContextMenu(event, contextMenuProps =>
|
||||
<MessageMenu
|
||||
message={message}
|
||||
channel={channel}
|
||||
onHeightUpdate={contextMenuProps.onHeightUpdate}
|
||||
/>
|
||||
);
|
||||
}
|
||||
});
|
|
@ -1,6 +0,0 @@
|
|||
# SearchReply
|
||||
|
||||
Adds a reply button to search results.
|
||||
|
||||
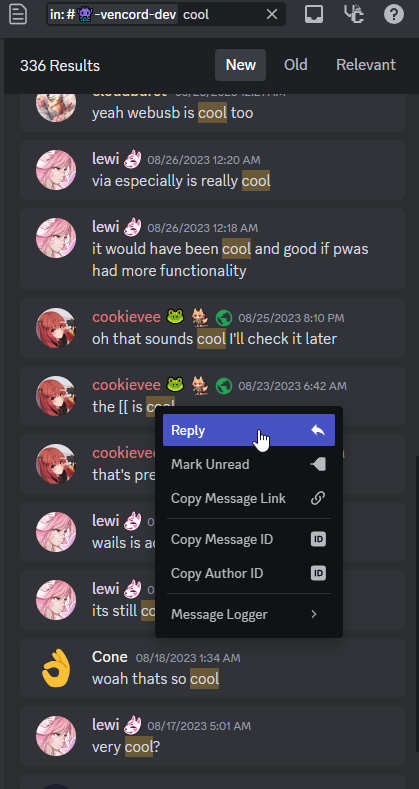
|
||||
|
|
@ -1,75 +0,0 @@
|
|||
/*
|
||||
* Vencord, a modification for Discord's desktop app
|
||||
* Copyright (c) 2023 Vendicated and contributors
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
import { findGroupChildrenByChildId, NavContextMenuPatchCallback } from "@api/ContextMenu";
|
||||
import { ReplyIcon } from "@components/Icons";
|
||||
import { Devs } from "@utils/constants";
|
||||
import definePlugin from "@utils/types";
|
||||
import { findByCodeLazy } from "@webpack";
|
||||
import { ChannelStore, i18n, Menu, PermissionsBits, PermissionStore, SelectedChannelStore } from "@webpack/common";
|
||||
import { Message } from "discord-types/general";
|
||||
|
||||
|
||||
const replyToMessage = findByCodeLazy(".TEXTAREA_FOCUS)", "showMentionToggle:");
|
||||
|
||||
const messageContextMenuPatch: NavContextMenuPatchCallback = (children, { message }: { message: Message; }) => {
|
||||
// make sure the message is in the selected channel
|
||||
if (SelectedChannelStore.getChannelId() !== message.channel_id) return;
|
||||
const channel = ChannelStore.getChannel(message?.channel_id);
|
||||
if (!channel) return;
|
||||
if (channel.guild_id && !PermissionStore.can(PermissionsBits.SEND_MESSAGES, channel)) return;
|
||||
|
||||
// dms and group chats
|
||||
const dmGroup = findGroupChildrenByChildId("pin", children);
|
||||
if (dmGroup && !dmGroup.some(child => child?.props?.id === "reply")) {
|
||||
const pinIndex = dmGroup.findIndex(c => c?.props.id === "pin");
|
||||
dmGroup.splice(pinIndex + 1, 0, (
|
||||
<Menu.MenuItem
|
||||
id="reply"
|
||||
label={i18n.Messages.MESSAGE_ACTION_REPLY}
|
||||
icon={ReplyIcon}
|
||||
action={(e: React.MouseEvent) => replyToMessage(channel, message, e)}
|
||||
/>
|
||||
));
|
||||
return;
|
||||
}
|
||||
|
||||
// servers
|
||||
const serverGroup = findGroupChildrenByChildId("mark-unread", children);
|
||||
if (serverGroup && !serverGroup.some(child => child?.props?.id === "reply")) {
|
||||
serverGroup.unshift((
|
||||
<Menu.MenuItem
|
||||
id="reply"
|
||||
label={i18n.Messages.MESSAGE_ACTION_REPLY}
|
||||
icon={ReplyIcon}
|
||||
action={(e: React.MouseEvent) => replyToMessage(channel, message, e)}
|
||||
/>
|
||||
));
|
||||
return;
|
||||
}
|
||||
};
|
||||
|
||||
|
||||
export default definePlugin({
|
||||
name: "SearchReply",
|
||||
description: "Adds a reply button to search results",
|
||||
authors: [Devs.Aria],
|
||||
contextMenus: {
|
||||
"message": messageContextMenuPatch
|
||||
}
|
||||
});
|
|
@ -148,7 +148,8 @@ function playSample(tempSettings: any, type: string) {
|
|||
const currentUser = UserStore.getCurrentUser();
|
||||
const myGuildId = SelectedGuildStore.getGuildId();
|
||||
|
||||
speak(formatText(settings[type + "Message"], currentUser.username, "general", (currentUser as any).globalName ?? currentUser.username, GuildMemberStore.getNick(myGuildId, currentUser.id) ?? currentUser.username), settings);
|
||||
const displayName = (currentUser as any).globalName ?? currentUser.username;
|
||||
speak(formatText(settings[type + "Message"], currentUser.username, "general", displayName, GuildMemberStore.getNick(myGuildId, currentUser.id) ?? displayName), settings);
|
||||
}
|
||||
|
||||
export default definePlugin({
|
||||
|
@ -179,7 +180,7 @@ export default definePlugin({
|
|||
const template = Settings.plugins.VcNarrator[type + "Message"];
|
||||
const user = isMe && !Settings.plugins.VcNarrator.sayOwnName ? "" : UserStore.getUser(userId).username;
|
||||
const displayName = user && ((UserStore.getUser(userId) as any).globalName ?? user);
|
||||
const nickname = user && (GuildMemberStore.getNick(myGuildId, userId) ?? user);
|
||||
const nickname = user && (GuildMemberStore.getNick(myGuildId, userId) ?? displayName);
|
||||
const channel = ChannelStore.getChannel(id).name;
|
||||
|
||||
speak(formatText(template, user, channel, displayName, nickname));
|
||||
|
|
Loading…
Reference in a new issue